
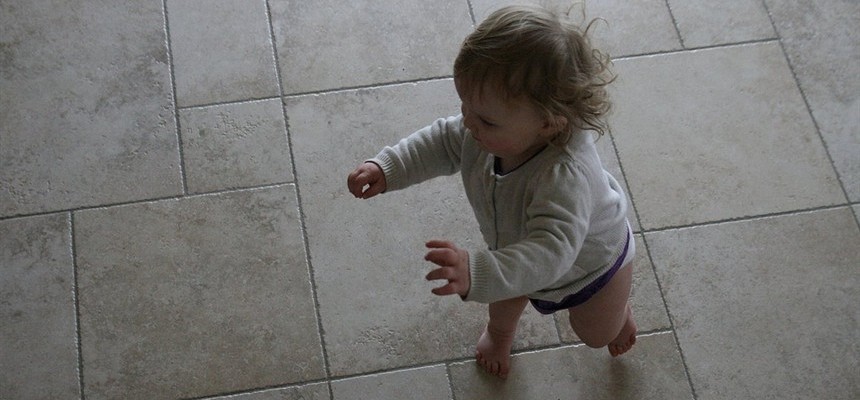
I'm unsure if this will trigger undefined behavior or not. Printf("dog size: %d\n", animal_size(&dog->animal)) Printf("dog size: %d\n", animal_size(&d2->animal)) Printf("dog addr: %p, d2 addr: %p\n", dog, d2) There will be cases when I want to know whether my animal is a dog, this is how I currently think of making an animal instance a dog, but I'm unsure if this will trigger undefined behavior or not. Typedef struct animal_vtable animal_vtable_t An upcast creates a base class reference. A downcast only succeeds if the objects are compatible types. Downcasting is the opposite going from a base class to a derived class, down the tree. What is upcasting and downcasting Upcasting is conversion from a derived (child) class to a base (parent) class. Imagine I have an animal struct with a vtable (meaning a struct of function pointers) and a dog like this: typedef void (*sound_func)(const animal_t *animal) Explicitly downcast to a subclass reference. The post C Introduction lists all of the C series of posts we have at this site.

One thing that comes to mind is how to correctly downcast a type in C without violating strict aliasing. use static_cast only if you are 100% sure the cast is good.I'm currently limited to coding in C and I want to do C object oriented programming. this means that when you have to downcast: Dereferencing sta_b has undefined behavior. BUT IT ISN'T // 'p' does not point to a 'B', so this is a bad cast! static_cast succeeds, resulting in // 'sta_b' being a BAD POINTER. No runtime check // sta_a will be identical to dyn_a aboveī* sta_b = static_cast(p) // static_cast assumes the cast is good. dynamic_cast will fail and dyn_b will be = nullptr // how static_cast worksĪ* sta_a = static_cast(p) // static_cast assumes the cast is good.

It will see that 'p' does not // point to a 'B', so this is a bad cast. dynamic_cast succeeds.ī* dyn_b = dynamic_cast(p) // dynamic_cast checks 'p'. Parent* p = &a // p points to an 'A' object // how dynamic cast works:Ī* dyn_a = dynamic_cast(p) // dynamic_cast checks 'p' to make sure it points to an 'A' // it does, so this cast is safe.
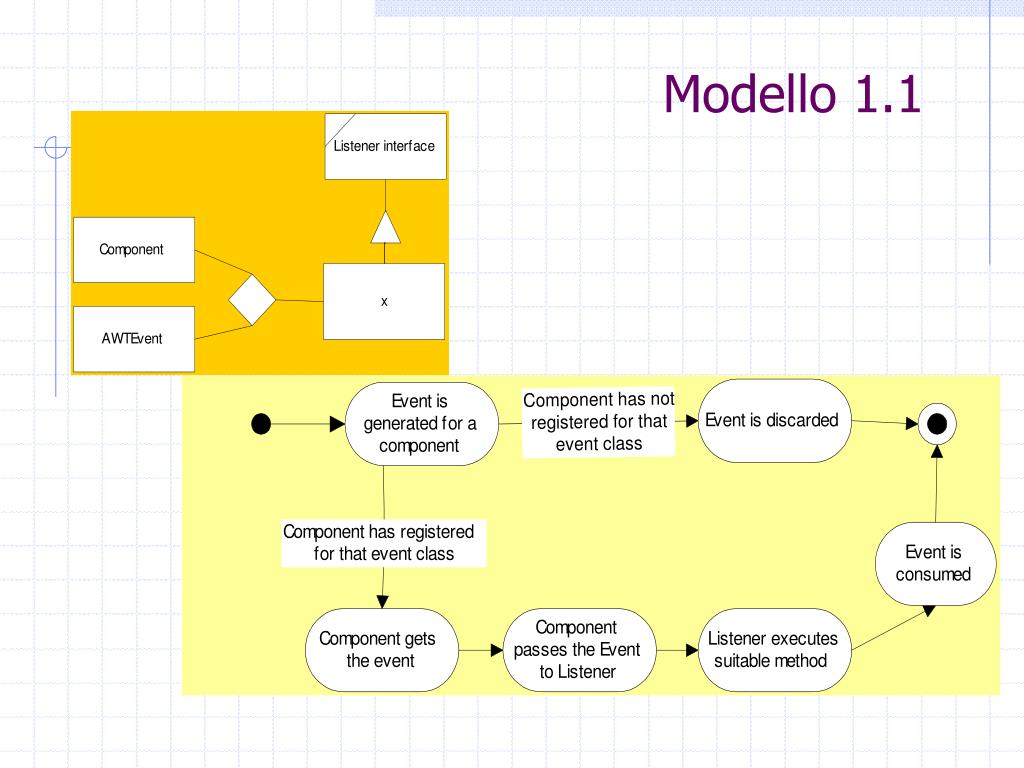
C++ allows that a derived class pointer (or reference) to be treated as a base class pointer. It can be achieved by using Polymorphism. Upcasting and downcasting give a possibility to build complicated programs with a simple syntax. But both casts cast the pointer the same way. Upcasting and downcasting are an important part of C++. The only difference is the runtime check. There is zero risk of a bad cast going unnoticed.ģ) dynamic_cast requires the class to be polymorphic. This means it's generally slower.Ģ) Also, because dynamic_cast does the runtime check, it's much safer. They are actually identical except for the below key points:ġ) dynamic_cast does a runtime check to make sure the cast is good. static_cast and dynamic_cast are quite simple. Really, you are way overcomplicating things here. As this behaviour is separate from the core conversion to numeric values, any errors raised during the downcasting will be surfaced regardless of the value of. static_cast will correctly adjust the vtable as needed. In this tutorial we will focus on Upcasting and Downcasting in C++, and since their use depends on Virtual Functions, we will be discussing those as well. Again whether or not it's "safe" has nothing to do with the sizeof() the class. dynamiccast is the preferred approach for a downcast or even better redesign to avoid needing them. Ensure any C style downcasts are replaced with at least a staticcast before refactoring further. A cast to a smaller integer type discards the most significant (left-most as youd write the full binary integer on paper) bits that are not.3 answers Top answer: DowncastsA cast to a smaller integer type discards the most significant (left-most as youd. The only time you should "always" use static_cast is if you know for a fact the cast will be safe. In conclusion NEVER use C style casts for downcasts. For example, you might cast a pointer to CMyDoc, called pMyDoc, to a pointer to CDocument using this expression: C++ Copy CDocument pDoc STATICDOWNCAST (CDocument, pMyDoc) If pMyDoc does not point to an object derived directly or indirectly from CDocument, the macro will ASSERT. B* b = static_cast(a) // compiler error, A and B are not related
